Our project is inspired by the commercial product, PicoDopp, which uses a similar scheme for foxhunting. Foxhunting is a contest where participants try to locate a transmitter, called the fox, simply by monitoring the signals it transmits.
Typically this is done using highly directional antennas — antennas that only receive signals from one direction. However, over short distances, especially within distances less than a mile or so, reflected signals can make these systems difficult to get accurate readings from. The only way to overcome this limitation is to use systems that do not rely on magnitude measurements.
We switch between antennas to simulate sinusoidal multiplication. We then measure certain frequencies our switching scheme injects into, which allows us to measure the phase difference between different antennas. Using the phase difference we can calculate path differences, and thus generate an estimate of where the signals are coming from.
We used multiple antennas to measure phase changes over different path lengths to get a bearing on a selected FM radio station. The image on the left shows the antenna we built for our project.
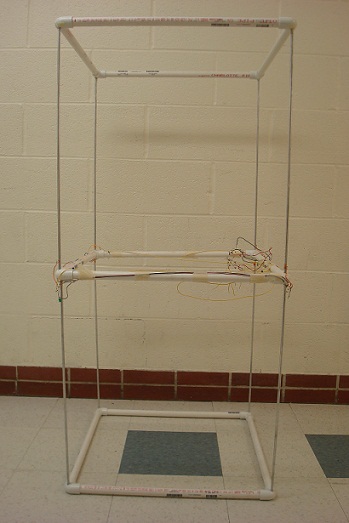
The antenna we built
Pre amplifier circuit and mux circuit hanging on our antenna
High Level Design
Logic Structure
So we are receiving 100 MHz antenna signals from our antennas, then the antenna signals are amplified by an analog circuit before they go into the mux. The outputs of the four muxes are combined and the combined output goes into the XOR multiplier. We use a Si 4840 radio chip as the receiver. Every time the control signal from the microcontroller will choose the output from one of the four muxes, and the chip will choose the correct frequency to multiply with the output from the mux block, and does the multiplication. Since we are only interested in frequencies at 1 KHz, we use a band pass amplifier to filter out the signals that we want, and pass this signal to the ADC to the microcontroller. The microcontroller also generates PWM signals which are the control signals to select which mux output will go into the receiver, and this performs the switching which is an essential step in simulating the doppler effect.
Here we try to show our logic structure using the following block diagram:
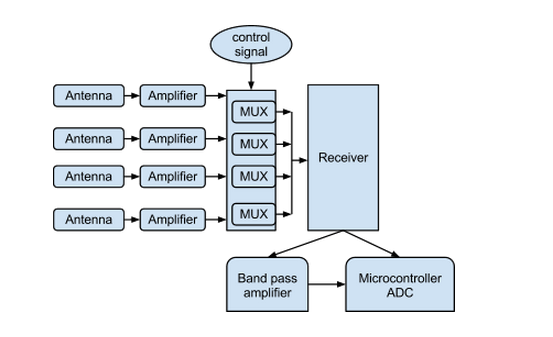
Logic structure of the system
Hardware/Software tradeoffs
There is a significant hardware/software trade off in our project. We are receiving 100 MHz signals from our antennas, and due to the high frequency of FM signals, it will be very difficult for us to deal with the signals using software due to the limitation of sampling frequency of the microcontroller. The microcontroller can not be sampled fast enough to meet our requirements of computation. Therefore, we introduce several analog circuits and a Si4840 radio chip into this project, which help us to perform filtering, amplifying, switching and multiplications of the signals. What’s more, we need to perform fourier transforms and this is hard to be done using analog circuits, therefore we switch to programming using microcontrollers instead of using analog circuits to perform filter transforms. We need to sample at at least 2 kHz in order to do the fourier analysis.
Hardware Design and Implementation
Hardware Setup
This figure shows our basic connections between the Si 4840 chip, Microcontroller and the LCD screen. The overall connection could be find here.
As shown above, we use function generator to power the Si4840 chip because the chip works at 2.0 to 3.6V.
When we were stuck in our project, we chose to switch to a more powerful RF receiver as shown above.
We use oscilloscope to monitor and debug for our project.
Hardware details
The antenna
The antenna was designed and built with the help of a friend of ours, Dana Hartsig. First, appropriate lengths of aluminum and PVC pipe were purchased and cut. We then connected them using *** Gap filling material ***. We sealed the joints with super glue.
To turn our device into four antennas that can be selected individually, we had to solder the included circuits to them. The amplifiers (shown below) were place as close to the antenna as possible to avoid any losses over the length of the wire. Then equal stretches of wire were connected to a central multiplexer (shown below). We needed equal wire lengths because different path lengths could lead to unwanted phase shifts on the signal.
The first amplifier comes between the antenna and the mux. After we receive the antenna signals, we use several yellow wires to connect the output of antenna with the mux circuits. Because we are going to join the outputs of the four muxes together, we keep the four muxes in the same area, relatively close to each other. The yellow wires from the antennas to the muxes may cause loss in the antenna signals which are originally weak, thus we introduce this analog amplifier to amplify the antenna signals. We use BT25A.215 in the following circuit:
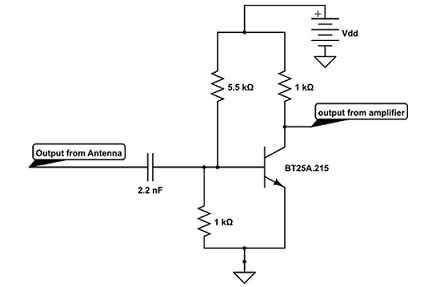
Pre amplifier
For this circuit, originally we used a diode between the gate of the BJT to the ground, and we tried to observe the output of the amplifier using the oscilloscope. However, we seldom get enough gain we need when we used the diode. Thus we began to use passive elements available in the lab for our testing and eventually we decide on this circuit based on trial and error.
Second comes the mux. For our project, we use a simple multplexer of our own design, shown in the figure below.
Mux Schematic.
The radio signal is connected to the dangling lead of the 1 nF capacitor. The control signals are generated by the microcontroller. Since there is a diode between the capacitor and the output of the mux, when the control signal is high, the intermediate node has a higher DC voltage than the output node, so the diode is turned on and the radio signal passes. When the control signal is low, the intermediate node has a lower DC voltage than the output node, so the diode is turned off and the radio signal does not pass. By setting the control signals high and low, we are simulating the effects of turning the switch on and off.
We are using Si 4840 as our receiver. The overall schematic shows the basic connections of this chip. We connected a separate crystal oscillator to help generate a stable reference signal. The antenna input is connected through a capacitor to remove any unwanted DC offset. The connections with the MCU are connected to a high voltage by pullup resistors as part of the I2C specifications.
Between the audio output of the FM receiver and the MCU is an analog filter. The filter’s most important purpose is removing signals higher than 4 kHz so we don’t violate sampling rate requirements. The design of the filter is done by plotting the transfer function in the mac grapher, and we decided on the values of the passive components used from the mac grapher. The result of plot is shown in figure below.We also used a simple button, connected by a pull-up resistor to the MCU.
Finally, we used an LCD. The connections can be found in the schematics.
Software Design and Implementation
Main function
Our program uses many states. We structured our project this way so as to avoid timing constraints. Our system’s “state machine” is actually just a constant flow from one state to another. Our system begins by initializing all of the peripheries required, including the LCD and the radio receiver. Then it enters the waiting state. Whenever a user presses the button, it moves on to the next state: measurements. There are eight different antenna switching and measurement patterns our system uses. We switch between every pair of antennas for six states, and then we rotate in a circle through the antennas going both ways for two more states. Finally, the system uses all of the data accrued during measurements to provide a reasonable estimate for the bearing of the transmitter.
Two wire interface
The radio receiver chip we used — the Si4840 — relies uses an I2C interface to communicate with the MCU. We send it a reset signal and wait for a second wire, called IRQ, to be pulled high to signal that the radio chip is ready to communicate.
I originally tried to use the Atmel1284P’s TWI hardware interface directly. However, I had difficulty debugging some of the errors my code produced. So I switched to Peter Fleury’s library which easily handled the basic interface protocol.
We send the radio chip instructions to start up, and tell it which frequency band to use. The radio chip selects the actual frequency it listens to based on the position of a potentiometer and the signal strength it can receive. If it can find a signal at the frequency the potentiometer has selected, it searches for more signal strength until it reaches band limits set by the microcontroller.
Once the radio chip has been initialized the microcontrollers starts regularly polling the radio circuit for changes in frequency, and waits for the user to indicate that it should continue on to the measurement phase.
Waiting on the button
Our button waiting code is very simple. It checks to see if a button is pressed once every 30 milliseconds. If the button is pressed for at least 60 milliseconds, then released for at least 60 milliseconds (to prevent repeated measurements) it begins a bearing calculation.
Measuring the signals
While measuring signals and switching antennas, the MCU enables OCR B interrupts. OCRB triggers shortly before OCRA to put the cpu to sleep so the control logic for the antennas take a consistent length of time.
We use the internal ADC to fill up eight arrays with 64 samples each. We sample at 8 kHz. Higher sampling frequencies will give better noise rejection, but they will also require more samples (and therefore more memory) to get enough cycles of a signal.
Calculating the bearings
The bearing calculations are a little more involved than the math discussed in the introductory math section discussed above. There are a few extra steps we have to go through in order to get a good estimate of where the radio station is coming from.
First, we need to calculate the noise offset. The signal amplitude from switching between the antennas on opposite corners of our antenna square should be precisely a factor of the square root of two larger than the signal amplitude from switching between the sides of the square. We use the square amplitude instead, so the square amplitude of the sides is twice the cross amplitude.
Most noise is generated from the original FM signal, as well as from imperfections in our switching system. Both of these effects add to all signals equally. This means, in the square sense, that the sides should have twice the error that the cross signals should have. We can adjust our internal guess at the error term until the two values are equal to get a reasonable estimate of the error.
Next we make a guess at the antenna angle. For this portion of the code we guess that the transmitter is in the direction of the antenna pairing that has the highest square magnitude. We then adjust our guess slightly by linearly interpolating between that antenna and the next antenna pairing with a large magnitude. This estimate will be accurate as long as the small signal approximation of sine and cosine holds. For our systems, this approximation leads to errors of ~4.5 degrees.
Finally, notice that two antennas alone cannot decide between two opposite directions. To make this final distinction we measure the phase of our received signal as we sweep our receiving antenna around in a circle. As the phase shift passes through zero we know that we have found the source of our signals. This measurement technique is not very accurate from an angle perspective — by sampling at 8 kHz we can only get 22.5 degree resolution — but when combined with the above method we can discriminate between two opposite directions.
Results
Performance
Usability
This design is very simple to use. A rotating potentiometer controls the frequency, which can be checked on the LCD. A button tells the device when to start a measurement.
Speed
The design ended up operating much faster than expected. We were worried it might take too long, because a number of floating point calculations are performed, but in the end the time required for all of the measurements and floating point calculations is barely perceivable.
Receiving Signal
We did not receive any FM signals. We believe the antenna was the weak link in our process. We measured the noise coming out of our antenna system, when all the antennas are off, as approximately 10 mV peak to peak. When the antenna system is turned on it rises to 20 mV peak to peak. This is a terrible signal-to-noise ratio, so it should not be that surprising that our radio chip was unable to find any radio stations.
Accuracy
Sadly, we were unable to verify the final working ability of the design because the antenna, a fundamental requirement of our design, did not function correctly. Though we were able to receive signals by connecting the antenna to a more powerful external receiver system, it is not clear that this receiver system responded to changes in antenna connections.
If I had a chance to try this again, I would try to find a commercial receiver system instead of trying to build my own from scratch. Then I would have had more time to focus on the core of the project.
Previous Work
Our project is based on similar designs, often referred to by the name PicoDopp, based on a commercial tool that uses the same kind of techniques our product uses.
We rely on FM specifications, though nothing we designed uses the FM standards directly.
Finally, communication with the radio receiver chip relies on the I2C protocol.
Failed Alternatives
For the amplifier between the antenna and the mux, at the beginning, we were thinking of using an amplifier to help us amply signals which is a very traditional way, but later on we discovered that we could not find suitable and affordable commercial amplifiers which operate in the frequency band that we need, which is 100MHz. After struggling for a while, we decide to design our own analog amplifier instead.
Originally we planned to use a commercial multiplexer, ADG904. Unfortunately, it proved to be too small to solder easily. We tried to use a SB300 PC breadboard as the base of the radio chip. We scratched the copper using sand paper where we needed our chip to sit on and glue the the chip upside down onto the breadboard. We then planned to solder wires from the pins on the chip to the breadboard. However, it turned out that even if we could solder wires to, for example five adjacent pins to the breadboard, the solder would melt when we tried to solder the wires onto the holes. We figured out later that this failure was caused because the holes we were trying to solder to were too close to the chip, which was approximately one width of our soldering gun away. Furthermore, due to the small size of the pins, we were forced to use very thin, fragile wires that broke easily.
We use a Si 4840 chip instead, which is much larger than the chips we originally planned to use. The Si 4840 chip is soldered onto the breakout board of its size and we could use Si 4840 instead. We replace the previous design with pre-packaged ICs to cut down on assembly time and any possible soldering errors.
Ethical and Legal Considerations
We closely followed IEEE Code of Ethics throughout our project. Specifically we ensured that safety precautions were considered and followed throughout our final project. Our project involved a huge amount of manual work, including soldering and assembling, and during the busy semester, we tried our best to make sure that we were not affecting the other students. Our original project idea was not realistic and we had realized this soon enough to find alternatives. This involved accurately portraying our current status and problems to our TAs and professor. We took time to explore the analog specifications, build and test the subcircuits. Besides, we had carried out intensive theoretical research in the relevant topic.
Background Math
Measuring the Direction of a Transmitter
FM radio signals have a primary “carrier wave”. This is basically just a pure sinusoid transmitted at some frequency f. Mathematically, this carrier wave can be expressed as:
where f is the frequency of carrier wave and t is the current time.
However, this signal is not quite the same at all points in space. Radio signals propagate at the speed of light. This means that an antenna listening to a frequency f placed a distance d further away from a radio source will experience a delay.
Delay can be expressed as a phase shift of the carrier wave:
d is the apparent change in distance between two antennas. The apparent distance can be calculated from D, the real distance between the two antennas, and Θ, the angle between the receiving antennas and the transmitter. Note that this equation assumes the transmitter is very far away compared with D.
Thus, θ can be expressed as a function of the real distance, D, the angle of the transmitter, ϕ, the frequency of interest, f, and the speed of light, c.
Given a pair of antennas, if we can measure this phase delay then we can determine the direction a transmitted signal is coming from.
Our project measures this phase delay using frequency mixing. Our goal is to add a signal at a certain frequency whose amplitude is proportional to the phase delay and is reduced to zero when the two signals experience no phase delay. We started with the identity:
This shows that a product of cosines produces a sum of cosines. Our final solution will require a lot of frequencies. We found the process easier to visualize with the help of Fourier Transforms:
We can now take the following product and sum:
And apply the identity above to get:
Now we can use the identity again to combine cosines of similar frequency to get:
Finally, we can pass this through a low frequency filter, and replace cosines with sines to get rid of the π to get:
So, by measuring the magnitude of this final signal we can measure the phase shift of the entire signal.
How this Applies to FM Signals
FM signals can be visualized as a central carrier frequency plus some audio information:
I should note, at this point, that modern FM signals are much more complicated. However, all the other signals contained in a typical FM broadcast are higher frequency still and thus can be safely ignored. I should also note that the main carrier wave is typically suppressed, but true suppression of the carrier is very difficult so it typically remains a significant portion of any FM broadcast.
To convert a broadcast FM signal to an audio signal, the FM signal is multiplied with a sinusoid of the same frequency as the original FM carrier, then passed through a low-pass filter to zero-center the signal:
This last signal is then converted from a frequency-modulated signal to an amplitude modulated signal and sent to an audio output.
Now we can incorporate our switching idea from earlier. If we modulate the signals from two antennas simultaneously we can inject a signal of our choosing:
Where the additional peaks in the final signal are proportional to the phase shift, as discussed in the previous section.
Switching Antennas is like multiplying by cosine
We do not actually multiply each antenna signal with a cosine shifted by a certain phase term, because creating and mixing that many signals would be expensive. Instead we turn each antenna on and off. This will inject more unwanted signals, but they will be on different frequencies.
To see this, consider the Fourier Series of a square wave:
square(x) = 12+4πcos(x)+2πcos(2x)+...
The second term is the cosine signal that we want. The other terms are undesired harmonies. However, a good filter system can remove these harmonies and leave us with the original signal we wanted.
The final antenna control signals are shown in the following image
This image shows a control signal for a single antenna. It is difficult to tell from this image. But note that on the left we are oscillating between two antennas. The duty cycle is 50%. In the middle the MCU is switching between four antennas, and the duty cycle drops to 25%.
Why not measure the FM signal directly
An astute reader might point out that the signals from each antenna are already different, and that if we have access to the signal from each antenna we should be able to measure the phase difference directly.
The problem is analog-to-digital converters and noise rejection. There are a lot of frequencies in the FM band — approximately one every MHz or more. Creating an ADC/filter system that can accurately measure a signal at 90 MHz, for example, but reject a signal at 91 MHz is very expensive and pushes the limit of what modern computers can do. Our switching system allows us to concentrate the high frequency circuitry in one good FM to audio converter and do the rest of our filtering and sampling at much lower frequencies.
Appendices
Schematic
Full schematics are available in PDF format.
Download the circuit schematic.
Code Listing
The system clock is set to 16 MHz with no fuse bits. The microcontroller is loaded on an ECE 476 prototype board. Further information can be found in the ATmega32 datasheet.
We are using the following libraries:
Peter Fleury's TWI controller-click here
LCD library files C file click here
Header file click here
Budget
Our budget was not to exceed $50. Our true out of pocket expenses was actually just $1.00 (for the mirrors). All other parts on this list were found in the lab or scavenged. We also chose to list the price of the acrylic parts as the cost it would take to fabricate it through Pololu, even though we used our own laser cutter.
Part | Quantity | Cost | Total | Source |
---|---|---|---|---|
5 foot PVC pipes | 4 |
$3 |
$12.0 |
Lowes |
PVC right angle turns | 12 |
$.25 |
$3.0 |
Lowes |
6 foot aluminum pipes | 4 |
$4.22 |
$16.88 |
McMasterrCarr |
Bottle of Sealant | 1 |
$3.0 |
$3.0 |
Lowes |
Si4840 | 1 |
$12.83 |
$12.83 |
Digikey |
BFT25A NPN transistor | 4 |
$0.67 |
$2.68 |
Digikey |
LCD | 1 |
$8 |
$8 |
ECE 476 Digital Lab |
200 kΩ potentiometer | 1 |
$0.86 |
$0.86 |
Digikey |
ATmega1284P with Protoboard | 1 |
-- |
-- |
ECE 476 Digital Lab |
LM358 Operational Amplifier | 1 |
-- |
-- |
ECE 476 Digital Lab |
Button | 1 |
-- |
-- |
Pre owned |
32786 Hz crystal oscillator | 1 |
-- |
-- |
ECE 476 Digital Lab |
diode | 4 |
-- |
-- |
ECE 476 Digital Lab |
5.5kΩ resistor | 8 |
-- |
-- |
ECE 476 Digital Lab |
1kΩ resistor | 21 |
-- |
-- |
ECE 476 Digital Lab |
100Ω resistor | 1 |
-- |
-- |
ECE 476 Digital Lab |
1.5kΩ resistor | 1 |
-- |
-- |
ECE 476 Digital Lab |
75 kΩ resistor | 1 |
-- |
-- |
ECE 476 Digital Lab |
2.2 nF capacitor | 6 |
-- |
-- |
ECE 476 Digital Lab |
1nF capacitor | 4 |
-- |
-- |
ECE 476 Digital Lab |
100 nF capacitor | 3 |
-- |
-- |
ECE 476 Digital Lab |
4.7nF | 1 |
-- |
-- |
ECE 476 Digital Lab |
22 pF capacitor | 3 |
-- |
-- |
Digikey |
10mF capacitor | 1 |
-- |
-- |
ECE 476 Digital Lab |
Total cost = 59.25
Tasks
Alexander Brady was the core programmer in this project who programmed the microcontroller and specified analog circuits. Peng Tian did the final design and implementation of all the analog circuits and connections, as well as soldered and assembed all the necessary components. Both teammates were present and helped with testing of the analog circuits as they were able.
References and Acknowledgements
Our final code used LCD controller code written by Ruibing Wang, modified by Bruce Land, as a starting point.
We used the LCD library created by Scienceprog.com. We used the TWI library created by Peter Fleury. Finally, we followed coding instructions found in the Si4822/26/40/44 Antenna, Schematic, Layout, and Design Guidelines.
We would like to thank Bruce Land for his assistance and providing the supplies in the lab. Thank you to our TAs who kept the lab open in the wee hours of the night and their invaluable advice.
Radio Receiver Datasheets
http://www.silabs.com/Support%20Documents/TechnicalDocs/AN602.pdf
http://www.silabs.com/Support%20Documents/TechnicalDocs/Si4840-44-A10.pdf
http://www.silabs.com/Support%20Documents/TechnicalDocs/AN610.pdf
Vendor
http://www.digikey.com/product-detail/en/SI4840-A10-GU/336-2153-ND/2709351
Transistor Datasheet
http://www.nxp.com/documents/data_sheet/BFT25A.pdf
Vendor
http://www.digikey.com/product-detail/en/BFT25A,215/568-1992-6-ND/1857795
Atmega1284p
http://people.ece.cornell.edu/land/courses/ece4760/AtmelStuff/mega1284full.pdf
(Provided by course instructor)
Background on Atmega1284p internal ADC
http://people.ece.cornell.edu/land/courses/ece4760/labs/f2012/lab3.html
Background on LCD
http://people.ece.cornell.edu/land/courses/ece4760/labs/f2012/lab1.html
(Provided by course instructor)
Background on Op Amp
http://people.ece.cornell.edu/land/courses/ece4760/labs/f2012/lab4.html
(Provided by course instructor)